List Union Functions
See also List Intersection, List Difference & List Symmetric Difference
Union of Two Lists
Function Syntax | (LM:ListUnion <l1> <l2>) |
Current Version | 1.0 |
Arguments | ||
---|---|---|
Symbol | Type | Description |
l1,l2 | List | Lists for which to return the Union |
Returns | ||
Type | Description | |
List | A list of all distinct items in the two lists |
Program Description
This subfunction will return a list expressing the union of two lists, that is, a list of all distinct items in the two supplied lists.
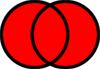
Recursive Version
Select all
;;---------------------=={ List Union }==---------------------;; ;; ;; ;; Returns a list expressing the union of two lists ;; ;;------------------------------------------------------------;; ;; Author: Lee Mac, Copyright © 2011 - www.lee-mac.com ;; ;;------------------------------------------------------------;; ;; Arguments: ;; ;; l1,l2 - Lists for which to return the union ;; ;;------------------------------------------------------------;; ;; Returns: A list of all distinct items in the two lists ;; ;;------------------------------------------------------------;; (defun LM:ListUnion ( l1 l2 ) ( (lambda ( f ) (f (append l1 l2))) (lambda ( l ) (if l (cons (car l) (f (vl-remove (car l) (cdr l)))))) ) )
Iterative Version
Select all
;;---------------------=={ List Union }==---------------------;; ;; ;; ;; Returns a list expressing the union of two lists ;; ;;------------------------------------------------------------;; ;; Author: Lee Mac, Copyright © 2011 - www.lee-mac.com ;; ;;------------------------------------------------------------;; ;; Arguments: ;; ;; l1,l2 - Lists for which to return the union ;; ;;------------------------------------------------------------;; ;; Returns: A list of all distinct items in the two lists ;; ;;------------------------------------------------------------;; (defun LM:ListUnion ( l1 l2 / x l ) (setq l1 (append l1 l2)) (while (setq x (car l1)) (setq l (cons x l) l1 (vl-remove x l1))) (reverse l) )
Example Function Call
_$ (LM:ListUnion '(1 2 3 4 5) '(2 4 6 8)) (1 2 3 4 5 6 8)
Union of a Set of Lists
Function Syntax | (LM:ListsUnion <l>) |
Current Version | 1.0 |
Arguments | ||
---|---|---|
Symbol | Type | Description |
l | List | A list of lists for which to return the union |
Returns | ||
Type | Description | |
List | A list of all distinct items in the set of lists |
Program Description
This subfunction will return a list expressing the union of a set of lists, that is, a list of all distinct items in every sublist in a supplied list.
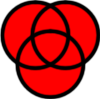
Recursive Version
Select all
;;---------------------=={ Lists Union }==--------------------;; ;; ;; ;; Returns a list expressing the union of a set of lists ;; ;;------------------------------------------------------------;; ;; Author: Lee Mac, Copyright © 2011 - www.lee-mac.com ;; ;;------------------------------------------------------------;; ;; Arguments: ;; ;; l - a list of lists for which to return the union ;; ;;------------------------------------------------------------;; ;; Returns: A list of distinct items in the set of lists ;; ;;------------------------------------------------------------;; (defun LM:ListsUnion ( l ) ( (lambda ( f ) (f (apply 'append l))) (lambda ( l ) (if l (cons (car l) (f (vl-remove (car l) (cdr l)))))) ) )
Iterative Version
Select all
;;---------------------=={ Lists Union }==--------------------;; ;; ;; ;; Returns a list expressing the union of a set of lists ;; ;;------------------------------------------------------------;; ;; Author: Lee Mac, Copyright © 2011 - www.lee-mac.com ;; ;;------------------------------------------------------------;; ;; Arguments: ;; ;; l - a list of lists for which to return the union ;; ;;------------------------------------------------------------;; ;; Returns: A list of distinct items in the set of lists ;; ;;------------------------------------------------------------;; (defun LM:ListsUnion ( l / x u ) (setq l (apply 'append l)) (while (setq x (car l)) (setq u (cons x u) l (vl-remove x l))) (reverse u) )
Example Function Call
_$ (LM:ListsUnion '((1 2 3 4 5) (1 3 5 7 9) (2 4 6 8))) (1 2 3 4 5 7 9 6 8)
See also List Intersection, List Difference & List Symmetric Difference